Having tried several Chinese building blocks in my power supply. I decided to use the “new” INA226 from TI. There are breakout boards available from the usual sources for almost nothing. A good reason to try one.
The INA226 breakout boards are capable of 3A and a maximum voltage of 36V. In case of a higher voltage there is an application note available from TI, to solve this problem. Another solution would be to put the shunt in the minus of your power supply.
I used the description of Giovanni Carera, adapted because it had a push-button and a SD card to write to.
A full description can be found HERE
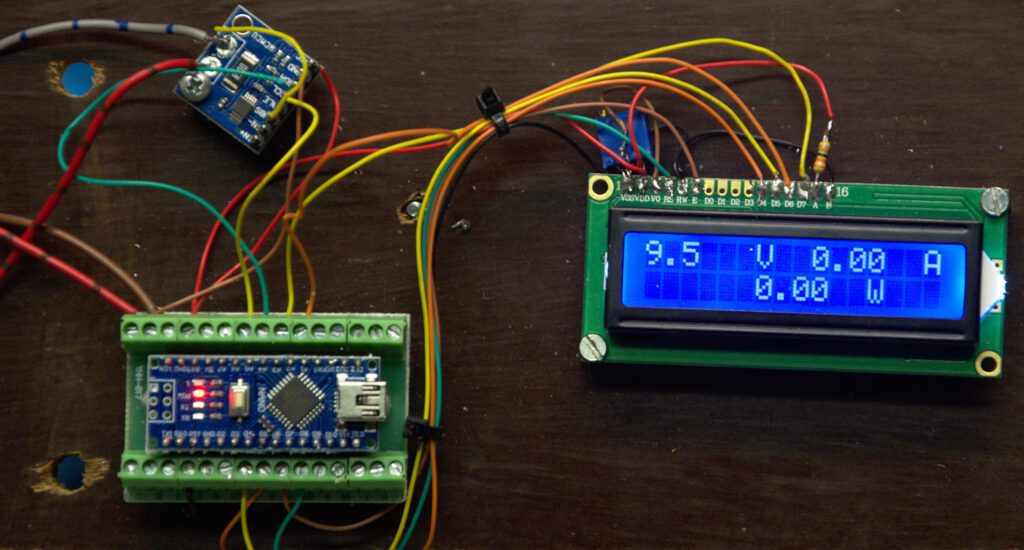
The adapted code for the current meter can be downloaded below.
/* Program ArduINA226 to current, voltage and power
with Arduino Nano and INA226 module
uses Korneliusz Jarzebski Library for INA226 (modified)
save measurements on SD if present
Giovanni Carrera, 14/03/2020
Modified (a little) Geert Stams PA3CSG 15 sept 2020
TNX ON6NL
*/
#include <LiquidCrystal.h>
#include <Wire.h>
#include <INA226.h>
// LCD pins
#define rs 7
#define en 6
#define d4 5
#define d5 4
#define d6 A2
#define d7 A3
// initialize the library by associating any needed LCD interface pin
LiquidCrystal lcd(rs, en, d4, d5, d6, d7);
INA226 ina;
char bline[17] = " ";// blank line
const int deltat= 200;// sampling period in milliseconds
unsigned long cmilli, pmilli;
boolean SDOk = true, FileHeader = true, ACQ = false;
unsigned int ns=0;
void setup() {
// set up the LCD's number of columns and rows:
lcd.begin(16, 2);
// Default INA226 address is 0x40
ina.begin(0x40);
lcd.setCursor ( 0 , 0);
lcd.print("INA226 PWR meter");
lcd.setCursor ( 3 , 1);
lcd.print(" PA3CSG");
delay (2000);
lcd.clear ();
ina.configure(INA226_AVERAGES_1, INA226_BUS_CONV_TIME_1100US, INA226_SHUNT_CONV_TIME_1100US, INA226_MODE_SHUNT_BUS_CONT);
// Set shunt resistance ann max current to Calibrate INA226. Example Rshunt = 0.1 ohm, Max expected current = 3 A
ina.calibrate(0.1, 1);
}
void loop() {
cmilli = millis();
if (cmilli - pmilli > deltat) {
pmilli = cmilli;
float volts = ina.readBusVoltage();
float current = ina.readShuntCurrent();
lcd.print(volts,3);
float power = ina.readBusPower();// INA calculate power
float Vshunt = ina.readShuntVoltage();
lcd.setCursor ( 0 , 0);
lcd.print(bline);
lcd.setCursor ( 0 , 0);
lcd.print(volts , 1);
lcd.setCursor ( 6 , 0);
lcd.print("V");
lcd.setCursor ( 9 , 0);
lcd.print(current , 2);
lcd.setCursor ( 15 , 0);
lcd.print("A");
lcd.setCursor ( 0 , 1);
lcd.print(bline);
lcd.setCursor ( 6 , 1);
lcd.print(power , 2);
lcd.setCursor ( 12 , 1);
lcd.print("W ");
}
}