To put my 23cm amplifier remote at the base of the tower I needed some kind of heating to prevent condensation. A dewpoint calculation was easily found on the web. Temperature and humidity measured by a DHT22
I added LCD readout and some switching for a solid state relay. Some of these relays require some current so you might have to add a driver relay / transistor.
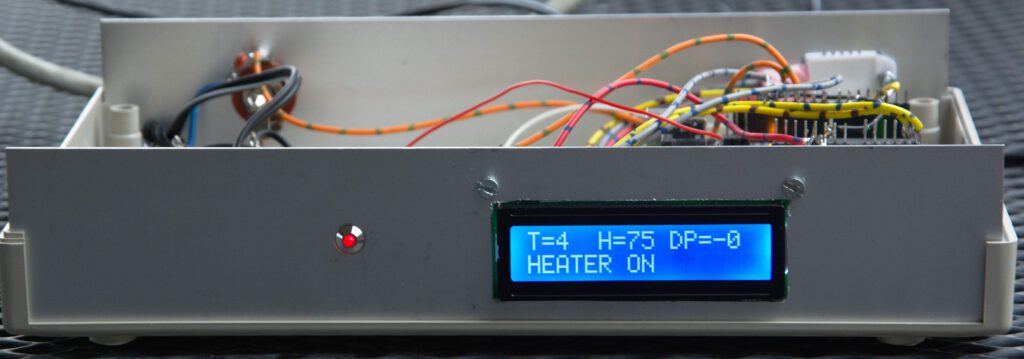
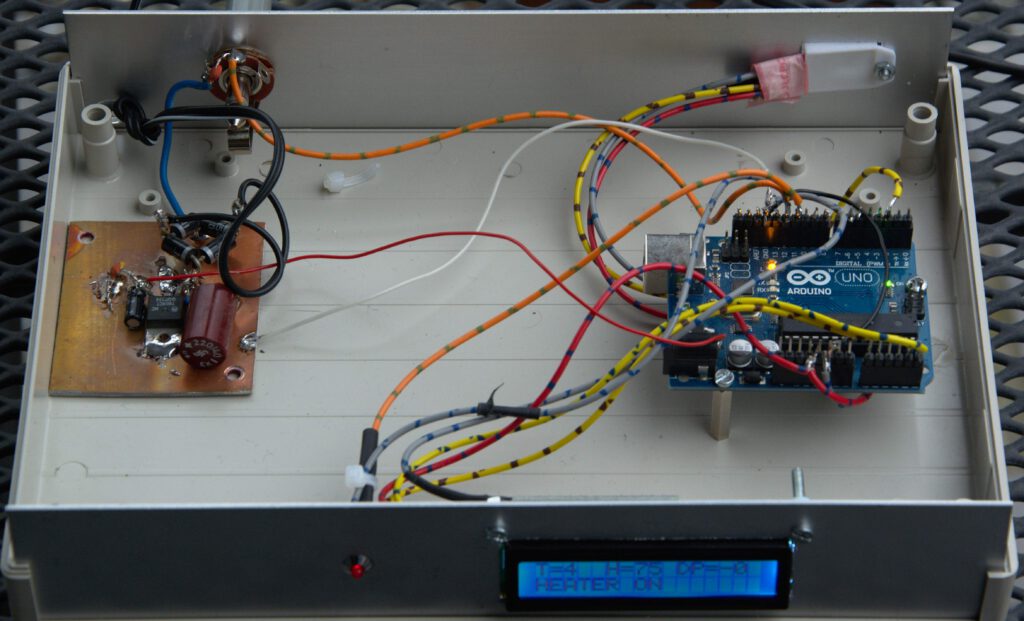
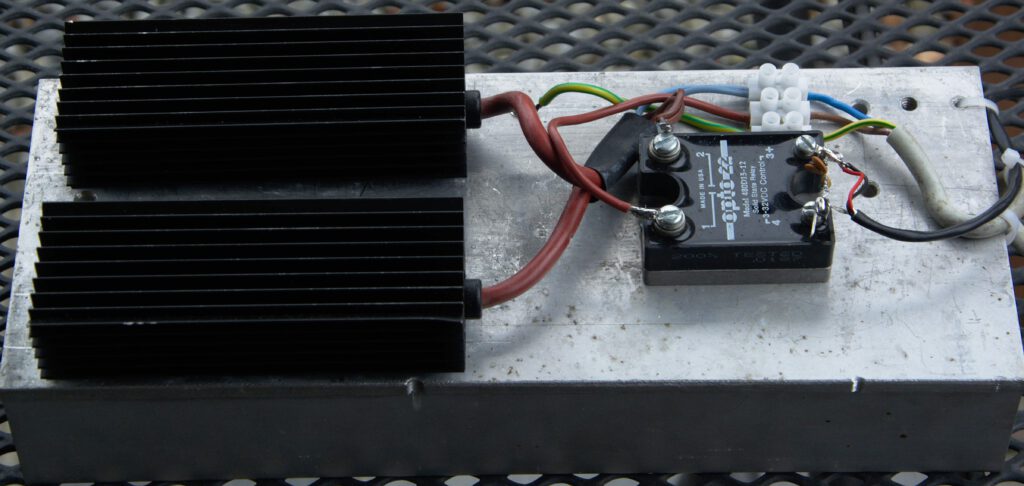
The code follows below:
// John Main added dewpoint code from : http://playground.arduino.cc/main/DHT11Lib
// Also added DegC output for Heat Index.
// dewPoint function NOAA
// reference (1) : http://wahiduddin.net/calc/density_algorithms.htm
// reference (2) : http://www.colorado.edu/geography/weather_station/Geog_site/about.htm
//
// januari 2021 Geert Stams, PA3CSG added LCD display and switching of a solid state relay to heat, preventing condensation.
// Pin 11 switches the led on
// Pin 12 drives the solid state realy
//
//******************************************************************************************************************************
//******************************************************************************************************************************
double dewPoint(double celsius, double humidity)
{
// (1) Saturation Vapor Pressure = ESGG(T)
double RATIO = 373.15 / (273.15 + celsius);
double RHS = -7.90298 * (RATIO - 1);
RHS += 5.02808 * log10(RATIO);
RHS += -1.3816e-7 * (pow(10, (11.344 * (1 - 1 / RATIO ))) - 1) ;
RHS += 8.1328e-3 * (pow(10, (-3.49149 * (RATIO - 1))) - 1) ;
RHS += log10(1013.246);
// factor -3 is to adjust units - Vapor Pressure SVP * humidity
double VP = pow(10, RHS - 3) * humidity;
// (2) DEWPOINT = F(Vapor Pressure)
double T = log(VP / 0.61078); // temp var
return (241.88 * T) / (17.558 - T);
}
// Example testing sketch for various DHT humidity/temperature sensors
// Written by ladyada, public domain
#include "DHT.h"
#include <Wire.h> // Library for I2C communication
#include <Wire.h> // Comes with Arduino IDE
#include <LiquidCrystal_I2C.h>
LiquidCrystal_I2C lcd(0x27, 2, 1, 0, 4, 5, 6, 7, 3, POSITIVE); // Set the LCD I2C address
#define DHTPIN 2 // what pin we're connected to
// Uncomment whatever type you're using!
//#define DHTTYPE DHT11 // DHT 11
#define DHTTYPE DHT22 // DHT 22 (AM2302)
//#define DHTTYPE DHT21 // DHT 21 (AM2301)
// Connect pin 1 (on the left) of the sensor to +5V
// NOTE: If using a board with 3.3V logic like an Arduino Due connect pin 1
// to 3.3V instead of 5V!
// Connect pin 2 of the sensor to whatever your DHTPIN is
// Connect pin 4 (on the right) of the sensor to GROUND
// Connect a 10K resistor from pin 2 (data) to pin 1 (power) of the sensor
// Initialize DHT sensor for normal 16mhz Arduino
DHT dht(DHTPIN, DHTTYPE);
// NOTE: For working with a faster chip, like an Arduino Due or Teensy, you
// might need to increase the threshold for cycle counts considered a 1 or 0.
// You can do this by passing a 3rd parameter for this threshold. It's a bit
// of fiddling to find the right value, but in general the faster the CPU the
// higher the value. The default for a 16mhz AVR is a value of 6. For an
// Arduino Due that runs at 84mhz a value of 30 works.
// Example to initialize DHT sensor for Arduino Due:
//DHT dht(DHTPIN, DHTTYPE, 30);
float DP = 0;
int Heatpin = 12;
int Ledpin = 11;
void setup() {
Serial.begin(9600);
Serial.println("DHTxx test!");
dht.begin();
lcd.begin(16,2); // initialize the lcd for 16 chars 2 lines, turn on backlight
}
void loop() {
// Wait a few seconds between measurements.
delay(5000);
// Reading temperature or humidity takes about 250 milliseconds!
// Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor)
float h = dht.readHumidity();
// Read temperature as Celsius
float t = dht.readTemperature();
// Read temperature as Fahrenheit
float f = dht.readTemperature(true);
// Check if any reads failed and exit early (to try again).
if (isnan(h) || isnan(t) || isnan(f)) {
Serial.println("Failed to read from DHT sensor!");
return;
}
// Compute heat index
// Must send in temp in Fahrenheit!
float hi = dht.computeHeatIndex(f, h);
float hiDegC = dht.convertFtoC(hi);
Serial.print("Humidity: ");
Serial.print(h);
Serial.print(" %\t");
Serial.print("Temperature: ");
Serial.print(t);
Serial.print(" *C ");
Serial.print(f);
Serial.print(" *F\t");
Serial.print("Heat index: ");
Serial.print(hiDegC);
Serial.print(" *C ");
Serial.print(hi);
Serial.print(" *F ");
Serial.print("Dew Point (*C): ");
Serial.println(dewPoint(t, h));
DP=(dewPoint(t, h));
lcd.setCursor(0,0);
lcd.print(" ");
lcd.setCursor(0,0);
lcd.print("T=");
lcd.setCursor(2,0);
lcd.print(t,0);
lcd.setCursor(5,0);
lcd.print("H=");
lcd.setCursor(7,0);
lcd.print(h,0);
lcd.setCursor(10,0);
lcd.print("DP=");
lcd.setCursor(13,0);
lcd.print(DP, 0);
if (t - DP <= 5)// Your temp has to be >3 degrees above the dewpoint
{
digitalWrite (Heatpin, HIGH);
digitalWrite (Ledpin, HIGH);
lcd.setCursor(0,1);
lcd.print("HEATER ON ");
}
else
{
digitalWrite (Heatpin, LOW);
digitalWrite (Ledpin, LOW);
lcd.setCursor(0,1);
lcd.print("HEATER OFF");
}
}